Imagine you have a web application that allows people view widgets by name. Somewhere deep in your codebase, a programmer has thoughtfully updated the existing ES5 SQL injection to this stylish new ES6 SQL injection:
Set-up the API endpoint through the.htaccess file; Instead of defining the API endpoint by code through dependency 'getpop/graphql-endpoint-for-wp' (and having to flush the rewrite rules), it can also be set-up with a rewrite rule in the.htaccess file. For this, remove that dependency from composer, and add the code below before the WordPress rewrite section (which starts with # BEGIN. Free React Decoupled Theme in WordPress using Gastby gatsby wordpress custom fields gatsby wordpress hosting gatsby wordpress gutenberg gatsby wordpr. In the WordPress database diagram represents how data is stored, so there is no 'beginning'. GraphQL, though, is an interface to retrieve data, hence there must be an initial stage from which to execute the query. This talk is a comparison of the REST API and GraphQL, and will help developers and stakeholders understand each tool in order to make an informed decision on which one to use. Search WordPress.tv WordCamps.
Injection attacks just like this one persist in-spite of the fact that a search for “sql injection tutorial” returns around 3,740,000 results on Google. They have made the top of the OWASP top 10 in 2010 and 2013 and probably will again in 2016 and likely for the foreseeable future. Motherboard even calls it “the hack that will never go away“.
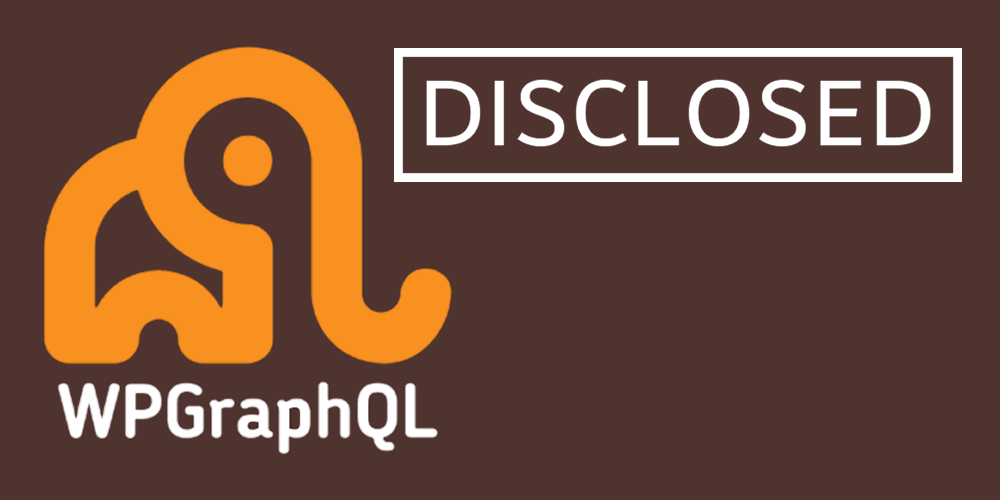
The standard answer to this sort of problem is input sanitization. It’s standard enough that it shows up in jokes.
But when faced with such consistent failure, it’s reasonable to ask if there isn’t something systemic going on.
Wordpress Graphql Authentication
There is a sub-field of security research known as Language Theoretic Security (Langsec) that is asking exactly that question.
Exploitation is unexpected computation caused reliably or probabilistically by some crafted inputs. — Meredith Patterson
Dropping the students table in the comic is exactly the kind of “unexpected computation” they are talking about. To combat it, Langsec encourages programmers to consider their inputs as a language and the sum of the adhoc checks on those inputs as a parser for that language.
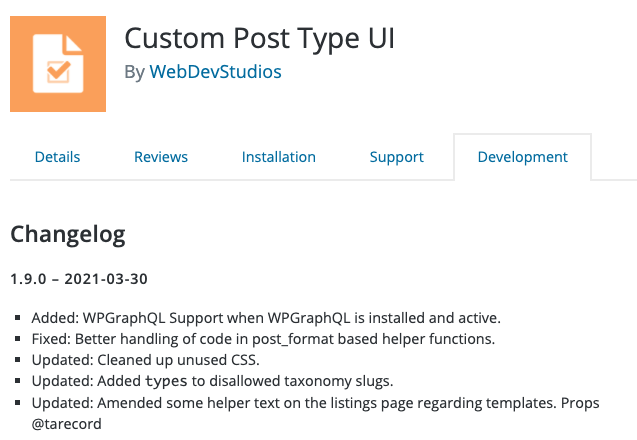
They advocate a clean separation of concerns between the recognition and processing of inputs, and bringing a recognizer of appropriate strength to bear on the input you are parsing (ie: not validating HTML/XML with a regex)
Where this starts intersecting with GraphQL is in what this actually implies:
This design and programming paradigm begins with a description of valid inputs to a program as a formal language (such as a grammar or a DFA). Flash for mac os x download. The purpose of such a disciplined specification is to cleanly separate the input-handling code and processing code.
A LangSec-compliant design properly transforms input-handling code into a recognizer for the input language; this recognizer rejects non-conforming inputs and transforms conforming inputs to structured data (such as an object or a tree structure, ready for type or value-based pattern matching).
The processing code can then access the structured data (but not the raw inputs or parsers’ temporary data artifacts) under a set of assumptions regarding the accepted inputs that are enforced by the recognizer.
If all that starts sounding kind of familiar, well it did to me too.
GraphQL

GraphQL allows you to define a formal language via it’s type system. As queries arrive, they are lexed, parsed, matched against the user defined types and formed into an Abstract Syntax Tree (AST). The contents of that AST are then made available to processing code via resolve functions.
The promise of Langsec is “software free from broad and currently dominant classes of bugs and vulnerabilities related to incorrect parsing and interpretation of messages between software components”, and GraphQL seems poised to put this within reach of every developer.
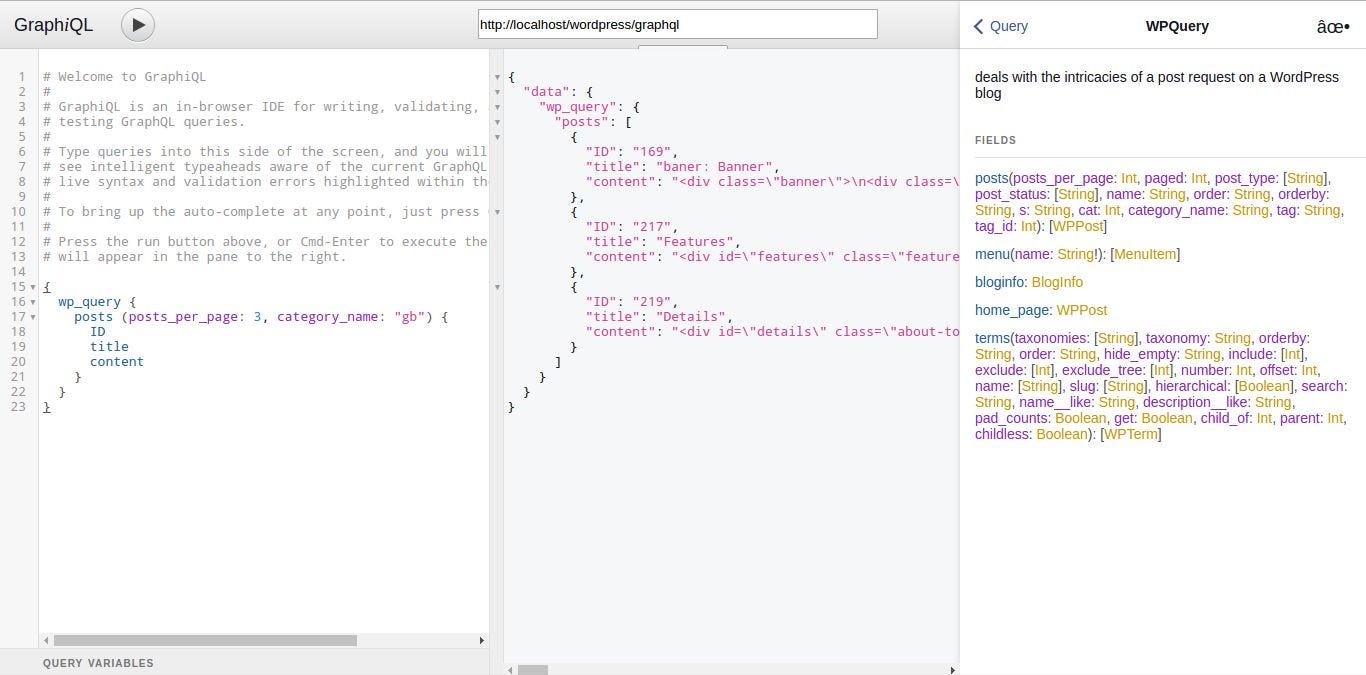
Turning back to the example we started with, how could we use GraphQL to protect against that SQL injection? Lets get this going in a test.
GraphQL brings lots of benefits (no need for API versioning, all data in a single round trip, etc…), and while those are compelling, simply passing strings into our backend systems misses an opportunity to do something different.
Let’s create a custom type that is more specific than just a “string”; an AlphabeticString.
Wordpress Graphql Nextjs
This test now passes; the SQL string is rejected during parsing before ever reaching the resolve function.
On making custom types
There are already libraries out there that provide custom types for things like URLs, datetime’s and other things, but you will definitely want to be able to make your own.
To start defining your own types you will need to understand the role
the various functions play:
The serialize function is the easiest to understand: GraphQL responses are serialized to JSON, and if this type needs special treatment before being included in a response, this is the place to do it.
Understanding parseValue
and parseLiteral
requires a quick look at two different queries: Nearest priority postboxes.
Wordpress Graphql 404
In the first query, name is a string literal, so your types parseLiteral
function will be called. The second query, the name is supplied as the value of a variable so parseValue
is called.
Your type could end up being used in either of those scenarios so it’s important to do your validation in both of those functions.
The standard GraphQL types (GraphQLInt, GraphQLFloat, etc.) also implement those same functions.
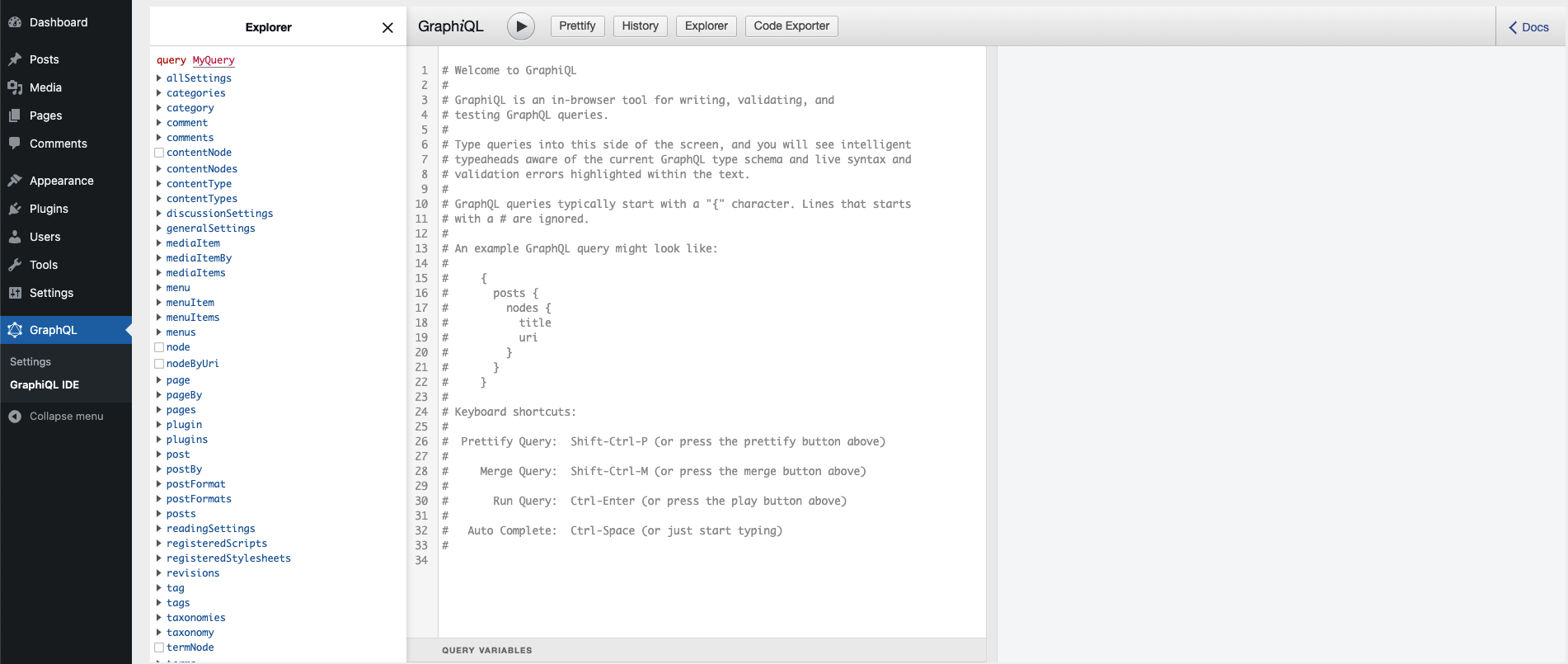
Putting the whole thing together, the process then looks something like this:
Wordpress Graphql Nuxt
A query arrives, gets tokenized, the parser builds the AST, calling parseValue
and parseLiteral
as needed. When the AST is complete, it recurses down the AST calling resolve, using parseValue
and parseLiteral
again on whatever is returned before calling serialize
on each to create the response.
Where to go from here
Langsec is a deep topic, with implications wider than just what is discussed here. While GraphQL is certainly not “Langsec in a box”, it not only seems to be making the design patterns that follow from Langsec’s insights a reality, it has a has a shot at making them mainstream. I’d love to see the Langsec lens turned on GraphQL and see how it can guide the evolution of the spec and the practices around it.
I would encourage you to dig into the ideas of Langsec, and the best place to start is here:
