- Cmake Visual Studio Code
- Cmake Visual Studio Code Generator
- Run Cmake In Visual Studio Code
- Cmake Tools Visual Studio Code
First written on 2020-09-11.
Last updated on 2021-01-02.
In this article and several more, I will be discussing developing a very simple C++ library and application using CMake and Visual Studio Code. I will also use git and Google Test, and port the project from Windows to Linux. Most of the information is applicable to using almost any IDE, or indeed, no IDE at all.
Why Use CMake?
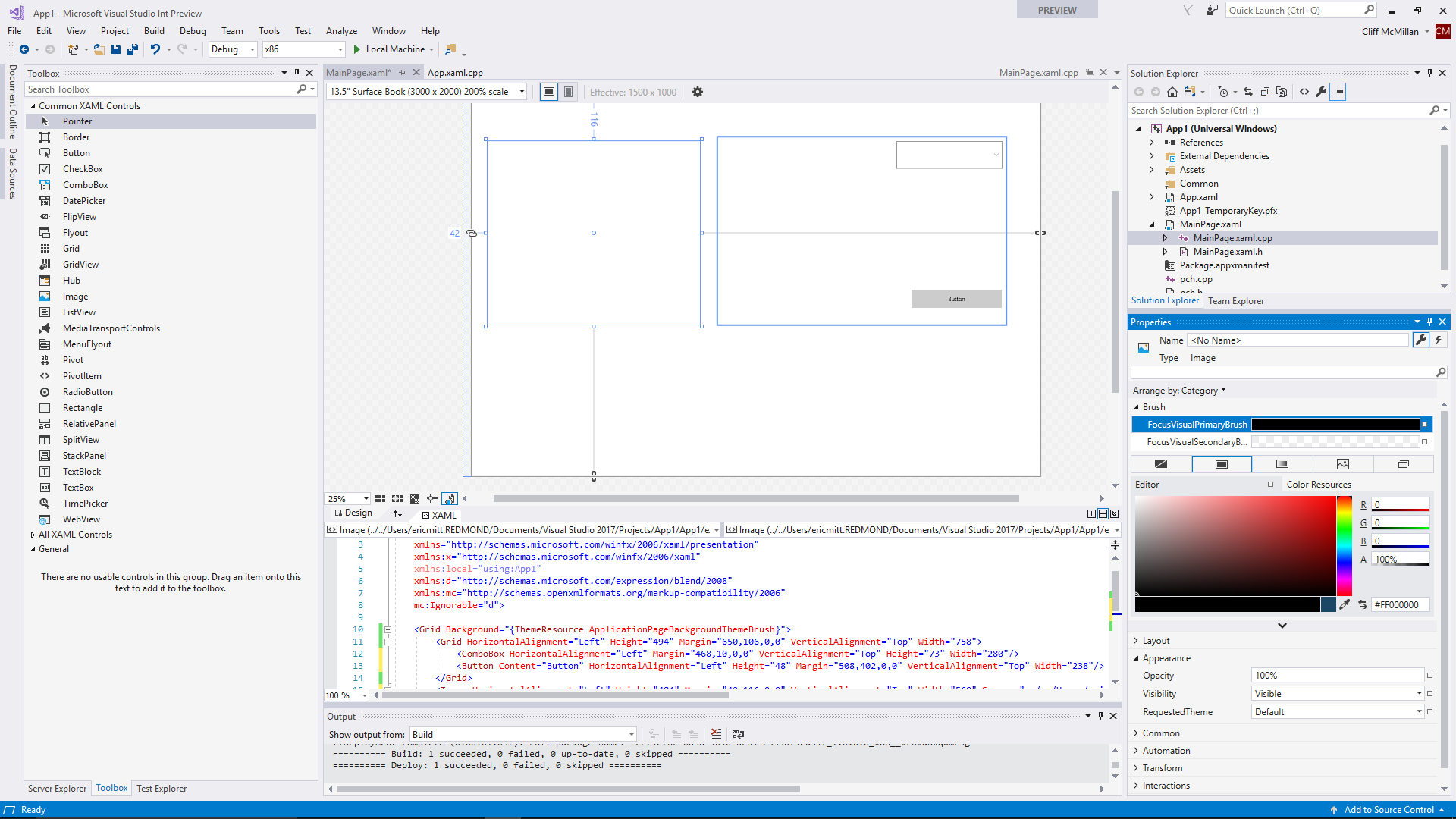
I want to use the CMake Tools extension for developing a CMake project in Visual Studio Code. I build the project in the command line with following command: PS projectbuild cmake -G'Visual Studio 14 2015 Win64' -DBOOSTROOT=somepath -DQTROOT=anotherpath projectpath. Using CMake to build #. OK, so now I knew how to build Visual Studio projects from command line. We had a pretty big C code base, that we wanted to build on Linux, macOS and Windows. CMake Tools for Visual Studio Code — CMake Tools 1.4.0 documentation CMake Tools for Visual Studio Code ¶ CMake Tools is an extension designed to make working with CMake-based projects as easy as possible. If you are new, check the Getting Started docs. Visual Studio Code First, install CMake Tools extension, because it conveniently does all the work of calling CMake with the right set of arguments for configure and build tasks. Open your project folder in Visual Studio Code, press Ctrl + Shift + P and run CMake: Configure. CMake is an open-source, cross-platform tool that uses compiler and platform independent configuration files to generate native build tool files specific to your compiler and platform. The CMake Tools extension integrates Visual Studio Code and CMake to make it easy to configure, build, and debug your C project.
CMake is a powerful and robust build system. You specify what you want done, not how to do it. CMake then takes that information and generates the files needed to build the system. For example, CMake can generate solution (.sln) and project files (.vcxproj) that Visual Studio and Visual Studio Code use on Windows. Similar capabilities are available for every other popular IDE. It can even create make files if you want to do everything from the command line. Because it can be called from the command line, it integrates well with continuous integration/continuous build systems.
You can specify the build tools that you want to use; for example, you can use MSVC or LLVM on Windows, and gnu or LLVM on Unix-like systems, including Linux, OSX, and MSYS or MinGW. Aside from specifying the tools to use, no other changes are required to the CMake specification files. You will see this when I port my project from Windows using the Visual Studio build tools to Linux using the gnu build tools.
With CMake, you can download, build, and use a large number of tools. I will show one example of this when I use Google Test in this project. Just about any tool that can be downloaded from the internet, and which provides CMake files for building, can be used.
Installing the Needed Tools
I will start the project on Windows using VS Code and the Visual Studio Build Tools, but if you wish, you can start with a different IDE, or even a different operating system. In a later article, I will discuss using the gnu tools on Linux (Ubuntu).
So let’s begin.
Installing Visual Studio Code and Extensions
On Windows, the latest version of Visual Studio Code is available on its download page. Select the appropriate version; click on the Windows button for the x64 version, or one of the ARM links for ARM if that is applicable to you. The download will begin. When it is completed, run the downloaded file.
Next, we need two VSCode extensions. Start VS Code and display the extensions panel (select View → Extensions from the main menu). In the search box, enter C++
. A number of C and C++ extensions are displayed. You want the one called C++. Make sure it is from Microsoft. This extension provides Intellisense, debugging, and browsing capabilities. Click on the Install button to install it.
The second extension is CMake Tools. Search for and install it.
Installing Visual Studio Build Tools
We need the build tools provided by Visual Studio. Don’t worry, we aren’t installing Visual Studio, just the build tools.
On the Visual Studio downloads page, move down into the All Downloads section. As I write this, the current version of Visual Studio is 2019, so I will be referring to it in this section. If a later version is available, use that instead. Select Tools for Visual Studio 2019. Click on the Download button for Build Tools for Visual Studio 2019. Download and save the file. When the download has completed, open the file. This starts Visual Studio Installer. Again, don’t worry, we are not installing Visual Studio, just the build tools. When the installer window opens select only the build tools. After some time (several minutes), the install will complete. Close the installer.
Open the Windows Start menu and start Developer Command Prompt for VS 2019; do not open the standard command prompt or Powershell. At the command prompt, enter:
The following should be displayed, although the version number may be different:
If the message says that it cannot find CMake, then the build tools did not install correctly.
You will almost always be starting VS Code from the command line of Developer Command Prompt, so you will probably want to add it to the Productivity section of the Start menu.
Installing Git
We will need git. If you have done any development work, you probably already have it installed. If not, Git for Windows is available here.
A Simple C++ Program With Library
We will start by creating a simple C++ program with a simple library. You can perform similar steps, with slight modifications, if you are on Linux or any other Unix-like system. To support the program, we will create a directory structure and start VS Code as follows:
Open a Developer Command Prompt. Enter:
In the Explorer list in VS Code, select the hello/include directory and create a new file called hello.h. Place the following code in that file and save it:
Again in the Explorer list, select hello/src and create a new file called hello.cpp. Place the following code in that file and save it:
Office 365 E5. Install Office mobile apps on up to five PCs or Macs, five tablets, and five phones per user. Make, receive, and transfer business calls from anywhere, using any device. Make informed decisions with data analytics and visualization. Safeguard your organization against malicious. Office 365 enterprise e5 cost.
Cmake Visual Studio Code
That is all the code we need for our library. Now create the program to use the library. In the Explorer list, select the apps directory and create a new file called main.cpp. Place the following code in that file and save it:
To build the library and program, we will use CMake. There are many examples of CMake on the internet, many of which are outdated or just plain bad. For this program and library, I am following Modern CMake by Henry Schreiner and others.
In the Explorer list, select VSCODE-CMAKE-HELLO in VS Code and create a new file. Call it CMakeLists.txt. Enter the following and save the file:
In the Explorer list, select apps and create a new file. Call it CMakeLists.txt. Enter the following and save the file:
Create a file called CMakeLists.txt in the hello directory and place the following code in it:
Using CMake With Visual Studio Code
Look at the status bar on the VS Code window. If it looks similar to this:
then terminate and restart VS Code. The status bar should now look like this:
From left to right, master*
indicates that you are editing the git master branch and that changes have been made. The symbols and 0s indicate that there are currently no errors in workspace. Next is a button that will run CMake (CMake: [Debug]
). No Kit Selected
indicates that the build tools have not yet been selected; we will get to them in a moment. Following that is a Build
button, the default target, a bug button that will run the application in debug mode, and a button that will run the application without starting the debugger. The remainder of the status bar provides other information that we are not currently concerned with.
We first have to run CMake to create the build files. Click on the CMake: [Debug]
button. The first time you do so, a list of build tool kits is displayed below the main menu. Select either Unspecified
, or Visual Studio Build Tools 2019 Release - amd64
. The No Kit Selected
text in the status bar will change to [Visual Studio Build Tools 2019 Release - amd64]
, and a list of CMake configurations are displayed: Debug
, Release
, MinSizeRel
, and RelWithDebInfo
.
Select Debug
. This will execute CMake and generate a Visual Studio solution file (.sln
) and Visual Studio project files (.vcxproj
) if there are no errors. If there are errors, then something is not right with the CMakeLists.txt files or the C++ source files.
If you selected any of the other CMake actions, the executable, library, and debug related files would be placed in other subdirectories. For example, if you build release versions, they will be placed in build/Release
.
The first time you run debugging by either clicking on the Bug
button, or by selecting Run → Start Debugging
, a list of build environments will be displayed just below the main menu. Select C++ (Windows)
.
To do a clean and rebuild, all we have to do is delete the build directory and all of its contents, then run CMake
and Build
.
Debugging
After a debug build, you can debug main.exe by clicking on the bug button in the status bar. Alternatively, you can select Run → Start Debugging
from the main menu. In the latter case, the first time you do this, a list of debug environments is displayed. Select C++ (Windows)
. If the active file in VS Code is a C++ source file, a list of configurations is then displayed. You can select either cl.exe - Build and debug active file
or Default configuration
. If a different type of file is active, such as CMakeLists.txt, then the configuration list is not displayed.
Cmake Visual Studio Code Generator
In either case, a file called launch.json is added to the .vscode directory. Open that file and change the program to ${workspaceFolder}/build/apps/Debug/main.exe
Now you can run debug from either the bug button or the menu item.
Summary and What’s Next
This article discussed how to create a C++ project containing a program called main and a library called hello with Visual Studio Code using CMake. How to debug the program is also discussed.
There is still much to do, but that will be discussed in the following articles:
- Adding GoogleTest to the project.
Run Cmake In Visual Studio Code
UPDATES
Cmake Tools Visual Studio Code
The code in hello.cpp was updated to correct a typo. Thanks Vlad T. for pointing that out.
