- Python Send A Text
- Python Message Queue Redis
- Python Imessage Software
- Python Imessage Tutorial
- Python Imessage Download
Source code:Lib/email/message.py
The central class in the email
package is the EmailMessage
class, imported from the email.message
module. It is the base class forthe email
object model. EmailMessage
provides the corefunctionality for setting and querying header fields, for accessing messagebodies, and for creating or modifying structured messages.
- We will use Python in this example, but you can follow a similar procedure for any of Voximplant’s other API client libraries - node.js, php,.NET, and Go. See here for more on integrating with Voximplant’s API clients. Python project setup. Voximplant’s Python API Client Library works with both Python 2.x and 3.x.
- If you have a Mac at home (like I do, it runs Kodi and is connected to my TV), you can also leverage it to control or query your home in different ways. Being an iPhone user, I thought it would be neat to be able to send and receive commands to my house using iMessages, whether I’m at home or somewhere else.
Py-imessage is a library to send iMessages from your Mac computer (it does not work on Windows/Linux). It was originally used to build an API for iMessages; however, Apple doesn’t support third-parties using iMessage over a few hundred marketing messages per day.
An email message consists of headers and a payload (which is also referredto as the content). Headers are RFC 5322 or RFC 6532 style field namesand values, where the field name and value are separated by a colon. The colonis not part of either the field name or the field value. The payload may be asimple text message, or a binary object, or a structured sequence ofsub-messages each with their own set of headers and their own payload. Thelatter type of payload is indicated by the message having a MIME type such asmultipart/* or message/rfc822.
The conceptual model provided by an EmailMessage
object is that of anordered dictionary of headers coupled with a payload that represents theRFC 5322 body of the message, which might be a list of sub-EmailMessage
objects. In addition to the normal dictionary methods for accessing the headernames and values, there are methods for accessing specialized information fromthe headers (for example the MIME content type), for operating on the payload,for generating a serialized version of the message, and for recursively walkingover the object tree.
The EmailMessage
dictionary-like interface is indexed by the headernames, which must be ASCII values. The values of the dictionary are stringswith some extra methods. Headers are stored and returned in case-preservingform, but field names are matched case-insensitively. Unlike a real dict,there is an ordering to the keys, and there can be duplicate keys. Additionalmethods are provided for working with headers that have duplicate keys.
The payload is either a string or bytes object, in the case of simple messageobjects, or a list of EmailMessage
objects, for MIME containerdocuments such as multipart/* and message/rfc822message objects.
email.message.
EmailMessage
(policy=default)¶If policy is specified use the rules it specifies to update and serializethe representation of the message. If policy is not set, use thedefault
policy, which follows the rules of the emailRFCs except for line endings (instead of the RFC mandated rn
, it usesthe Python standard n
line endings). For more information see thepolicy
documentation.
as_string
(unixfrom=False, maxheaderlen=None, policy=None)¶Return the entire message flattened as a string. When optionalunixfrom is true, the envelope header is included in the returnedstring. unixfrom defaults to False
. For backward compatibilitywith the base Message
class maxheaderlen isaccepted, but defaults to None
, which means that by default the linelength is controlled by themax_line_length
of the policy. Thepolicy argument may be used to override the default policy obtainedfrom the message instance. This can be used to control some of theformatting produced by the method, since the specified policy will bepassed to the Generator
.
Flattening the message may trigger changes to the EmailMessage
if defaults need to be filled in to complete the transformation to astring (for example, MIME boundaries may be generated or modified).
Note that this method is provided as a convenience and may not be themost useful way to serialize messages in your application, especially ifyou are dealing with multiple messages. Seeemail.generator.Generator
for a more flexible API forserializing messages. Note also that this method is restricted toproducing messages serialized as “7 bit clean” whenutf8
is False
, which is the default.
Changed in version 3.6: the default behavior when maxheaderlenis not specified was changed from defaulting to 0 to defaultingto the value of max_line_length from the policy.
__str__
()¶Equivalent to as_string(policy=self.policy.clone(utf8=True))
. Allowsstr(msg)
to produce a string containing the serialized message in areadable format.
Changed in version 3.4: the method was changed to use utf8=True
,thus producing an RFC 6531-like message representation, instead ofbeing a direct alias for as_string()
.
as_bytes
(unixfrom=False, policy=None)¶Return the entire message flattened as a bytes object. When optionalunixfrom is true, the envelope header is included in the returnedstring. unixfrom defaults to False
. The policy argument may beused to override the default policy obtained from the message instance.This can be used to control some of the formatting produced by themethod, since the specified policy will be passed to theBytesGenerator
.
Flattening the message may trigger changes to the EmailMessage
if defaults need to be filled in to complete the transformation to astring (for example, MIME boundaries may be generated or modified).
Note that this method is provided as a convenience and may not be themost useful way to serialize messages in your application, especially ifyou are dealing with multiple messages. Seeemail.generator.BytesGenerator
for a more flexible API forserializing messages.
__bytes__
()¶Equivalent to as_bytes()
. Allows bytes(msg)
to produce abytes object containing the serialized message.
is_multipart
()¶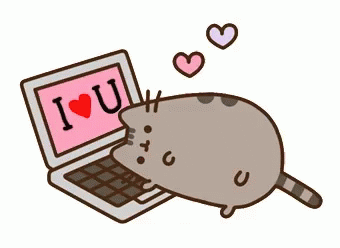
Return True
if the message’s payload is a list ofsub-EmailMessage
objects, otherwise return False
. Whenis_multipart()
returns False
, the payload should be a stringobject (which might be a CTE encoded binary payload). Note thatis_multipart()
returning True
does not necessarily mean that“msg.get_content_maintype() ‘multipart’” will return the True
.For example, is_multipart
will return True
when theEmailMessage
is of type message/rfc822
.
set_unixfrom
(unixfrom)¶Set the message’s envelope header to unixfrom, which should be astring. (See mboxMessage
for a brief description ofthis header.)
get_unixfrom
()¶Return the message’s envelope header. Defaults to None
if theenvelope header was never set.
The following methods implement the mapping-like interface for accessing themessage’s headers. Note that there are some semantic differencesbetween these methods and a normal mapping (i.e. dictionary) interface. Forexample, in a dictionary there are no duplicate keys, but here there may beduplicate message headers. Also, in dictionaries there is no guaranteedorder to the keys returned by keys()
, but in an EmailMessage
object, headers are always returned in the order they appeared in theoriginal message, or in which they were added to the message later. Anyheader deleted and then re-added is always appended to the end of theheader list.
These semantic differences are intentional and are biased towardconvenience in the most common use cases.
Note that in all cases, any envelope header present in the message is notincluded in the mapping interface.
__len__
()¶Return the total number of headers, including duplicates.
__contains__
(name)¶Return True
if the message object has a field named name. Matching isdone without regard to case and name does not include the trailingcolon. Used for the in
operator. For example:
__getitem__
(name)¶Return the value of the named header field. name does not include thecolon field separator. If the header is missing, None
is returned; aKeyError
is never raised.
Note that if the named field appears more than once in the message’sheaders, exactly which of those field values will be returned isundefined. Use the get_all()
method to get the values of all theextant headers named name.
Using the standard (non-compat32
) policies, the returned value is aninstance of a subclass of email.headerregistry.BaseHeader
.
__setitem__
(name, val)¶Add a header to the message with field name name and value val. Thefield is appended to the end of the message’s existing headers.
Note that this does not overwrite or delete any existing header with the samename. If you want to ensure that the new header is the only one present in themessage with field name name, delete the field first, e.g.:
If the policy
defines certain headers to be unique (as the standardpolicies do), this method may raise a ValueError
when an attemptis made to assign a value to such a header when one already exists. Thisbehavior is intentional for consistency’s sake, but do not depend on itas we may choose to make such assignments do an automatic deletion of theexisting header in the future.
__delitem__
(name)¶Delete all occurrences of the field with name name from the message’sheaders. No exception is raised if the named field isn’t present in theheaders.
keys
()¶Return a list of all the message’s header field names.
values
()¶Return a list of all the message’s field values.
items
()¶Return a list of 2-tuples containing all the message’s field headers andvalues.
get
(name, failobj=None)¶Return the value of the named header field. This is identical to__getitem__()
except that optional failobj is returned if thenamed header is missing (failobj defaults to None
).
Here are some additional useful header related methods:
get_all
(name, failobj=None)¶Return a list of all the values for the field named name. If there areno such named headers in the message, failobj is returned (defaults toNone
). Mac wont download adobe.
add_header
(_name, _value, **_params)¶Extended header setting. This method is similar to __setitem__()
except that additional header parameters can be provided as keywordarguments. _name is the header field to add and _value is theprimary value for the header.
For each item in the keyword argument dictionary _params, the key istaken as the parameter name, with underscores converted to dashes (sincedashes are illegal in Python identifiers). Normally, the parameter willbe added as key='value'
unless the value is None
, in which caseonly the key will be added.
If the value contains non-ASCII characters, the charset and language maybe explicitly controlled by specifying the value as a three tuple in theformat (CHARSET,LANGUAGE,VALUE)
, where CHARSET
is a stringnaming the charset to be used to encode the value, LANGUAGE
canusually be set to None
or the empty string (see RFC 2231 for otherpossibilities), and VALUE
is the string value containing non-ASCIIcode points. If a three tuple is not passed and the value containsnon-ASCII characters, it is automatically encoded in RFC 2231 formatusing a CHARSET
of utf-8
and a LANGUAGE
of None
.
Here is an example:
This will add a header that looks like
An example of the extended interface with non-ASCII characters:
replace_header
(_name, _value)¶Replace a header. Replace the first header found in the message thatmatches _name, retaining header order and field name case of theoriginal header. If no matching header is found, raise aKeyError
.
get_content_type
()¶Return the message’s content type, coerced to lower case of the formmaintype/subtype. If there is no Content-Typeheader in the message return the value returned byget_default_type()
. If the Content-Type header isinvalid, return text/plain
.
(According to RFC 2045, messages always have a default type,get_content_type()
will always return a value. RFC 2045 definesa message’s default type to be text/plain unless it appearsinside a multipart/digest container, in which case it wouldbe message/rfc822. If the Content-Type headerhas an invalid type specification, RFC 2045 mandates that the defaulttype be text/plain.)
get_content_maintype
()¶Return the message’s main content type. This is the maintypepart of the string returned by get_content_type()
.
get_content_subtype
()¶Return the message’s sub-content type. This is the subtypepart of the string returned by get_content_type()
.
get_default_type
()¶Return the default content type. Most messages have a default contenttype of text/plain, except for messages that are subparts ofmultipart/digest containers. Such subparts have a defaultcontent type of message/rfc822.
set_default_type
(ctype)¶Set the default content type. ctype should either betext/plain or message/rfc822, although this isnot enforced. The default content type is not stored in theContent-Type header, so it only affects the return value ofthe get_content_type
methods when no Content-Typeheader is present in the message.
set_param
(param, value, header='Content-Type', requote=True, charset=None, language=', replace=False)¶Set a parameter in the Content-Type header. If theparameter already exists in the header, replace its value with value.When header is Content-Type
(the default) and the header does notyet exist in the message, add it, set its value totext/plain, and append the new parameter value. Optionalheader specifies an alternative header to Content-Type.
If the value contains non-ASCII characters, the charset and language maybe explicitly specified using the optional charset and languageparameters. Optional language specifies the RFC 2231 language,defaulting to the empty string. Both charset and language should bestrings. The default is to use the utf8
charset and None
forthe language.
If replace is False
(the default) the header is moved to theend of the list of headers. If replace is True
, the headerwill be updated in place.
Use of the requote parameter with EmailMessage
objects isdeprecated.
Note that existing parameter values of headers may be accessed throughthe params
attribute of theheader value (for example, msg['Content-Type'].params['charset']
).
Changed in version 3.4: replace
keyword was added.
del_param
(param, header='content-type', requote=True)¶Remove the given parameter completely from the Content-Typeheader. The header will be re-written in place without the parameter orits value. Optional header specifies an alternative toContent-Type.
Use of the requote parameter with EmailMessage
objects isdeprecated.
get_filename
(failobj=None)¶Return the value of the filename
parameter of theContent-Disposition header of the message. If the headerdoes not have a filename
parameter, this method falls back to lookingfor the name
parameter on the Content-Type header. Ifneither is found, or the header is missing, then failobj is returned.The returned string will always be unquoted as peremail.utils.unquote()
.
get_boundary
(failobj=None)¶Return the value of the boundary
parameter of theContent-Type header of the message, or failobj if eitherthe header is missing, or has no boundary
parameter. The returnedstring will always be unquoted as per email.utils.unquote()
.
set_boundary
(boundary)¶Python Send A Text
Set the boundary
parameter of the Content-Type header toboundary. set_boundary()
will always quote boundary ifnecessary. A HeaderParseError
is raised if themessage object has no Content-Type header.
Note that using this method is subtly different from deleting the oldContent-Type header and adding a new one with the newboundary via add_header()
, because set_boundary()
preservesthe order of the Content-Type header in the list ofheaders.
get_content_charset
(failobj=None)¶Return the charset
parameter of the Content-Type header,coerced to lower case. If there is no Content-Type header, or ifthat header has no charset
parameter, failobj is returned.
get_charsets
(failobj=None)¶Return a list containing the character set names in the message. If themessage is a multipart, then the list will contain one elementfor each subpart in the payload, otherwise, it will be a list of length 1.
Each item in the list will be a string which is the value of thecharset
parameter in the Content-Type Download bamboo software for mac. header for therepresented subpart. If the subpart has no Content-Typeheader, no charset
parameter, or is not of the text mainMIME type, then that item in the returned list will be failobj.
is_attachment
()¶Return True
if there is a Content-Disposition headerand its (case insensitive) value is attachment
, False
otherwise.
Changed in version 3.4.2: is_attachment is now a method instead of a property, for consistencywith is_multipart()
.
get_content_disposition
()¶Return the lowercased value (without parameters) of the message’sContent-Disposition header if it has one, or None
. Thepossible values for this method are inline, attachment or None
if the message follows RFC 2183.
The following methods relate to interrogating and manipulating the content(payload) of the message.
walk
()¶The walk()
method is an all-purpose generator which can be used toiterate over all the parts and subparts of a message object tree, indepth-first traversal order. You will typically use walk()
as theiterator in a for
loop; each iteration returns the next subpart.
Python Message Queue Redis
Here’s an example that prints the MIME type of every part of a multipartmessage structure:
walk
iterates over the subparts of any part whereis_multipart()
returns True
, even thoughmsg.get_content_maintype()'multipart'
may return False
. Wecan see this in our example by making use of the _structure
debughelper function:
Python Imessage Software
Here the message
parts are not multiparts
, but they do containsubparts. is_multipart()
returns True
and walk
descendsinto the subparts.
get_body
(preferencelist=('related', 'html', 'plain'))¶Return the MIME part that is the best candidate to be the “body” of themessage.
preferencelist must be a sequence of strings from the set related
,html
, and plain
, and indicates the order of preference for thecontent type of the part returned.
Start looking for candidate matches with the object on which theget_body
method is called.
If related
is not included in preferencelist, consider the rootpart (or subpart of the root part) of any related encountered as acandidate if the (sub-)part matches a preference.
When encountering a multipart/related
, check the start
parameterand if a part with a matching Content-ID is found, consideronly it when looking for candidate matches. Otherwise consider only thefirst (default root) part of the multipart/related
.
If a part has a Content-Disposition header, only considerthe part a candidate match if the value of the header is inline
.
If none of the candidates matches any of the preferences inpreferencelist, return None
.
Notes: (1) For most applications the only preferencelist combinationsthat really make sense are ('plain',)
, ('html','plain')
, and thedefault ('related','html','plain')
. (2) Because matching startswith the object on which get_body
is called, calling get_body
ona multipart/related
will return the object itself unlesspreferencelist has a non-default value. (3) Messages (or message parts)that do not specify a Content-Type or whoseContent-Type header is invalid will be treated as if theyare of type text/plain
, which may occasionally cause get_body
toreturn unexpected results.
iter_attachments
()¶Return an iterator over all of the immediate sub-parts of the messagethat are not candidate “body” parts. That is, skip the first occurrenceof each of text/plain
, text/html
, multipart/related
, ormultipart/alternative
(unless they are explicitly marked asattachments via Content-Disposition: attachment), andreturn all remaining parts. When applied directly to amultipart/related
, return an iterator over the all the related partsexcept the root part (ie: the part pointed to by the start
parameter,or the first part if there is no start
parameter or the start
parameter doesn’t match the Content-ID of any of theparts). When applied directly to a multipart/alternative
or anon-multipart
, return an empty iterator.
iter_parts
()¶Return an iterator over all of the immediate sub-parts of the message,which will be empty for a non-multipart
. (See alsowalk()
.)
get_content
(*args, content_manager=None, **kw)¶Call the get_content()
methodof the content_manager, passing self as the message object, and passingalong any other arguments or keywords as additional arguments. Ifcontent_manager is not specified, use the content_manager
specifiedby the current policy
.
set_content
(*args, content_manager=None, **kw)¶Call the set_content()
methodof the content_manager, passing self as the message object, and passingalong any other arguments or keywords as additional arguments. Ifcontent_manager is not specified, use the content_manager
specifiedby the current policy
.
make_related
(boundary=None)¶Convert a non-multipart
message into a multipart/related
message,moving any existing Content- headers and payload into a(new) first part of the multipart
. If boundary is specified, useit as the boundary string in the multipart, otherwise leave the boundaryto be automatically created when it is needed (for example, when themessage is serialized).
make_alternative
(boundary=None)¶Convert a non-multipart
or a multipart/related
into amultipart/alternative
, moving any existing Content-headers and payload into a (new) first part of the multipart
. Ifboundary is specified, use it as the boundary string in the multipart,otherwise leave the boundary to be automatically created when it isneeded (for example, when the message is serialized).
make_mixed
(boundary=None)¶Convert a non-multipart
, a multipart/related
, or amultipart-alternative
into a multipart/mixed
, moving any existingContent- headers and payload into a (new) first part of themultipart
. If boundary is specified, use it as the boundary stringin the multipart, otherwise leave the boundary to be automaticallycreated when it is needed (for example, when the message is serialized).
add_related
(*args, content_manager=None, **kw)¶If the message is a multipart/related
, create a new messageobject, pass all of the arguments to its set_content()
method,and attach()
it to the multipart
. Ifthe message is a non-multipart
, call make_related()
and thenproceed as above. If the message is any other type of multipart
,raise a TypeError
. If content_manager is not specified, usethe content_manager
specified by the current policy
.If the added part has no Content-Disposition header,add one with the value inline
.
add_alternative
(*args, content_manager=None, **kw)¶If the message is a multipart/alternative
, create a new messageobject, pass all of the arguments to its set_content()
method, andattach()
it to the multipart
. If themessage is a non-multipart
or multipart/related
, callmake_alternative()
and then proceed as above. If the message isany other type of multipart
, raise a TypeError
. Ifcontent_manager is not specified, use the content_manager
specifiedby the current policy
.
add_attachment
(*args, content_manager=None, **kw)¶If the message is a multipart/mixed
, create a new message object,pass all of the arguments to its set_content()
method, andattach()
it to the multipart
. If themessage is a non-multipart
, multipart/related
, ormultipart/alternative
, call make_mixed()
and then proceed asabove. If content_manager is not specified, use the content_manager
specified by the current policy
. If the added parthas no Content-Disposition header, add one with the valueattachment
. This method can be used both for explicit attachments(Content-Disposition: attachment) and inline
attachments(Content-Disposition: inline), by passing appropriateoptions to the content_manager
.
clear
()¶Remove the payload and all of the headers.
clear_content
()¶Remove the payload and all of the Content-
headers, leavingall other headers intact and in their original order.
EmailMessage
objects have the following instance attributes:
preamble
¶The format of a MIME document allows for some text between the blank linefollowing the headers, and the first multipart boundary string. Normally,this text is never visible in a MIME-aware mail reader because it fallsoutside the standard MIME armor. However, when viewing the raw text ofthe message, or when viewing the message in a non-MIME aware reader, thistext can become visible.
The preamble attribute contains this leading extra-armor text for MIMEdocuments. When the Parser
discovers some textafter the headers but before the first boundary string, it assigns thistext to the message’s preamble attribute. When theGenerator
is writing out the plain textrepresentation of a MIME message, and it finds themessage has a preamble attribute, it will write this text in the areabetween the headers and the first boundary. See email.parser
andemail.generator
for details.
Note that if the message object has no preamble, the preamble attributewill be None
.
epilogue
¶The epilogue attribute acts the same way as the preamble attribute,except that it contains text that appears between the last boundary andthe end of the message. As with the preamble
,if there is no epilog text this attribute will be None
.
defects
¶The defects attribute contains a list of all the problems found whenparsing this message. See email.errors
for a detailed descriptionof the possible parsing defects.
email.message.
MIMEPart
(policy=default)¶This class represents a subpart of a MIME message. It is identical toEmailMessage
, except that no MIME-Version headers areadded when set_content()
is called, since sub-parts donot need their own MIME-Version headers.
Footnotes
Python Imessage Tutorial
Python Imessage Download
Originally added in 3.4 as a provisional module. Docs for legacy message class moved toemail.message.Message: Representing an email message using the compat32 API.
